ERC20 Token işlemleri
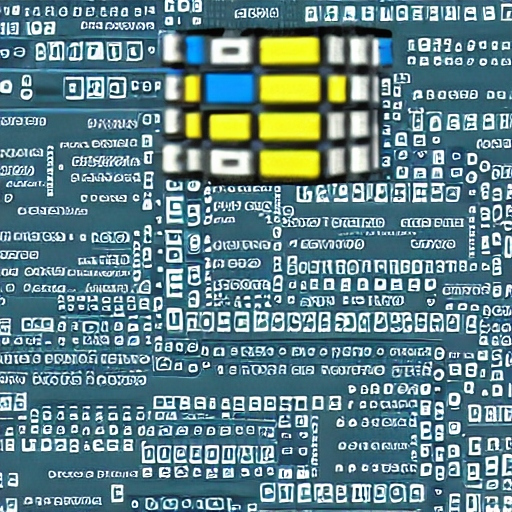
Ethereum ERC20 token’larının bakiyesini gösteren ve bu token’ları başka bir adrese transfer eden bir Node.js kod parçacığı yazalım ve beraber inceleyelim.
const Web3 = require('web3');
const Tx = require('ethereumjs-tx').Transaction;
const contractAddress = '0xc770eefad204b5180df6a14ee197d99d808ee52d'; // Token'ın adresi
const privateKey = '0xe5cb2c278909272a12e1eee4d3f4e75f279a2dfdc951bcbb45926a18e96770e2'; // Transfer yapacak adresin private key'i
const fromAddress = '0xE96Bb2A8535FD608A056c6cdBeA44f9B0Fa0a405'; // Transfer yapacak adres
const toAddress = '
0x68cbbbd43589e792c577a18050c1a3ee8caad10b'; // Token'ların gönderileceği adres
const tokenAmount = 10; // Transfer edilecek token miktarı
// ABI'si dosyadan okuma, ABI bir SmartContrat'ın Interface'idir.
// Kontratınızı deploy ettiğinizde Remix ide üzerinden size verilir.
const abi = require('./abi.json');
// Web3 provider oluşturma
// Infura.io dan bir hesap oluşturup buna uygun linki aşağıya giriniz.
const web3 = new Web3(new Web3.providers.HttpProvider('https://mainnet.infura.io/v3/...'));
// Contract instance oluşturma
const contract = new web3.eth.Contract(abi, contractAddress);
// Bakiyeyi kontrol etme fonksiyonu
async function checkBalance(fromAddress) {
try {
// balanceOf() fonksiyonunun çağrılması
const balance = await contract.methods.balanceOf(fromAddress).call();
console.log(`${fromAddress} adresindeki ERC20 token bakiyeniz : ${balance}`);
} catch (error) {
console.log(`Error: ${error}`);
}
}
// Token transfer etme fonksiyonu
async function transfer(fromAddress, toAddress, tokenAmount, privateKey) {
try {
// nonce hesaplama
const nonce = await web3.eth.getTransactionCount(fromAddress);
// gas price hesaplama
const gasPrice = await web3.eth.getGasPrice();
// gas limit
const gasLimit = 21000;
// value
const value = 0;
// data
const data = contract.methods.transfer(toAddress, tokenAmount).encodeABI();
// raw transaction oluşturma
const rawTx = {
nonce,
gasPrice,
gasLimit,
to: contractAddress,
value,
data
};
// transaction oluşturma
const tx = new Tx(rawTx);
// transaction imzalama
tx.sign(Buffer.from(privateKey.slice(2), 'hex'));
// serialize edilmiş transaction
const serializedTx = tx.serialize();
// signed transaction yollama
await web3.eth.sendSignedTransaction('0x' + serializedTx.toString('hex'))
.on('transactionHash', (hash) => {
console.log(`Transaction hash : ${hash}`)
})
.on('receipt', (receipt) => {
console.log(`Transaction receipt : ${JSON.stringify(receipt)}`)
})
.on('confirmation', (confirmationNumber, receipt) => {
console.log(`Transaction confirmation : ${confirmationNumber} = ${JSON.stringify(receipt)}`)
})
}
catch (err){
console.log(err);
}
}
// Bakiyeyi alalım.
await checkBalance(fromAddress);
// Transferi yapalım.
await transfer(fromAddress, toAddress, tokenAmount, privateKey)
Bu kod parçacığı fromAddress
adresindeki ERC20 token’ların bakiyesini konsola yazdırır ve sonrasında toAddress
adresine 10 token transfer eder. Lütfen dikkatli olun çünkü privateKey
adresin tüm yetkinliğidir. Paylaşırken içinde gerçek private key’ini içermelidir ve bunu herhangi bir yerde paylaşmamak önemlidir.
Bakiye sorgusu işlemi READ işlemi olduğu için bir transaction fee si ödenmez. Fakat transfer işleminde bir bakiye değişimi (giriş ve çıkış) olur ve bu blocklara yazılır. Yazma işleminin ve bu bakiye hesaplama işlemin bir maliyeti olduğu için bu işlemde bir transaction fee si ödenir. Mevcut ethereum sisteminde fee ler sadece eth olarak ödenir.